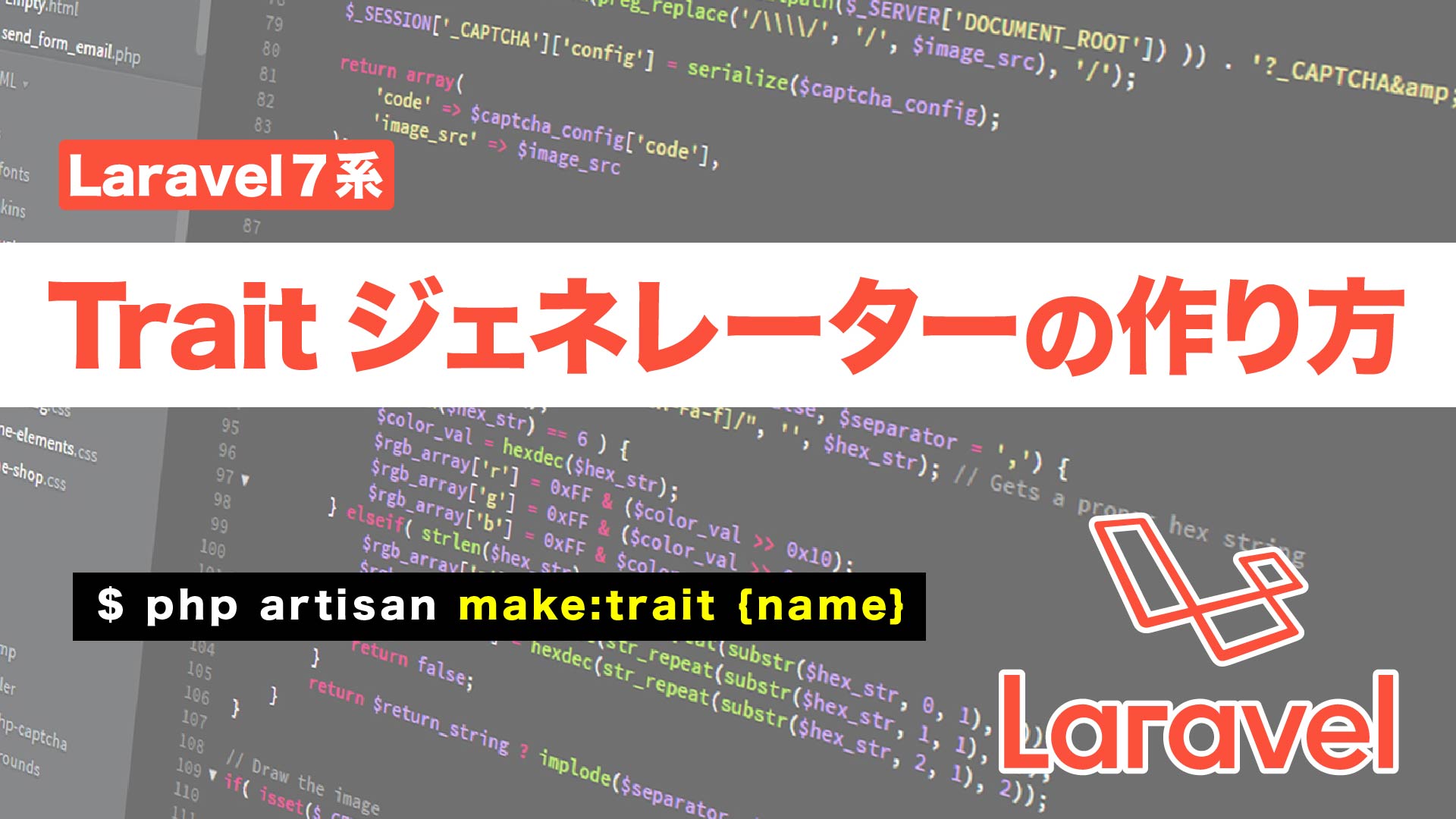
Laravel7がリリースされて、さっそくそれを使った開発の機会がやってきましたので、使用頻度の高いものをまとめていきたいと思います。
5系や6系の情報は多く出回っていますが、6系がLTSということもあって7系の情報は少なめですね。
今回は「Laravel7系でTraitのmakeコマンドを作成する方法」をご紹介します。
・環境
PHP:7.2.5
Laravel:7.18.0
・参考ページ
Traitジェネレーターの作成
Traitジェネレーターを作成するためには、以下の3ステップで行います。
- コマンドの準備
- テンプレート(.stub)の準備
- Traitの作成
では手順に沿って説明していきます。
コマンドの準備
コントローラーやリクエストと同じようにmakeコマンドでトレイトを作成するためには、トレイト用のmakeコマンドを作成しなければいけません。
まずはCommandクラスを用意するために、Laravelディレクトリで以下のコマンドを実行してください。
$ php artisan make:command TraitMakeCommand
これで、以下のディレクトリにTraitMakeCommand.phpが作成されます。
app/Console/Commands
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class TraitMakeCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'command:name';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
//
}
}
コマンド実行直後のTraitMakeCommand.phpは上記のような内容になっていますので、以下のように書き換えてください。
<?php
namespace App\Console\Commands;
use Illuminate\Console\GeneratorCommand as Command;
class TraitMakeCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'make:trait {name}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Create a new trait';
/**
* The type of class being generated.
*
* @var string
*/
protected $type = 'Trait';
/**
* Get the stub file for the generator.
*
* @return string
*/
protected function getStub()
{
base_path('/stubs/trait.stub');
}
/**
* Get the default namespace for the class.
*
* @param string $rootNamespace
* @return string
*/
protected function getDefaultNamespace($rootNamespace)
{
return $rootNamespace.'\Traits';
}
}
※Laravel5〜6系では$signatureに入れているコマンド名の{name}が不要だったのですが、こちらが抜けているとmake:traitを実行しても「The “name” argument does not exist.」のエラーを吐かれたので7系では追加しました
テンプレート(.stub)の準備
書き換えたTraitMakeCommand.php内に記述しているように、テンプレート(.stub)のファイルを以下のディレクトリへ用意します。
Laravelルート/stubs
※Laravel7系からはphp artisan stub:publishでRequestやControllerなど標準で搭載されているmakeコマンド用のstubがカスタムできるようになったため、その格納先と同じくLaravel直下のstubsディレクトリを保存先としています
trait.stubというファイル名で作成し、以下のように記述してください。
<?php
namespace {{ namespace }};
trait {{ class }}
{
//
}
これでテンプレートファイルの準備は完了です。
Traitの作成
コマンドとテンプレートが準備できれば、正常にコマンドが登録されているか、listコマンドを使って確認してみてください。
$ php artisan list | grep make
make
make:channel Create a new channel class
make:command Create a new Artisan command
make:controller Create a new controller class
make:event Create a new event class
make:exception Create a new custom exception class
make:factory Create a new model factory
make:job Create a new job class
make:listener Create a new event listener class
make:mail Create a new email class
make:middleware Create a new middleware class
make:migration Create a new migration file
make:model Create a new Eloquent model class
make:notification Create a new notification class
make:observer Create a new observer class
make:policy Create a new policy class
make:provider Create a new service provider class
make:request Create a new form request class
make:resource Create a new resource
make:rule Create a new validation rule
make:seeder Create a new seeder class
make:test Create a new test class
make:trait Create a new trait
最終行にmake:traitという記述があれば正常に作成されています。
コマンドが作成されたら、make:traitを使ってトレイトを作成します。
$php artisan make:trait TestTrait
正常に終了すれば、TestTrait.phpが追加されているはずです。
app/Traits/TestTrait.php
<?php
namespace App\Traits;
trait TestTrait
{
//
}
これでLaravel7系でトレイト用のmakeコマンドを作成することができました。
まとめ
いかがだったでしょうか。
今回は「Laravel7系でTraitのmakeコマンドを作成する方法」をご紹介しました。
他にも自作でmakeコマンドを作成したい人は、同じやり方でできますので試してみてください。
Laravel7系の情報は5や6と比べるとまだまだ少ないですので、もしこれからLaravel7系を使った開発を考えている人は、ぜひ参考にしてくださいね。
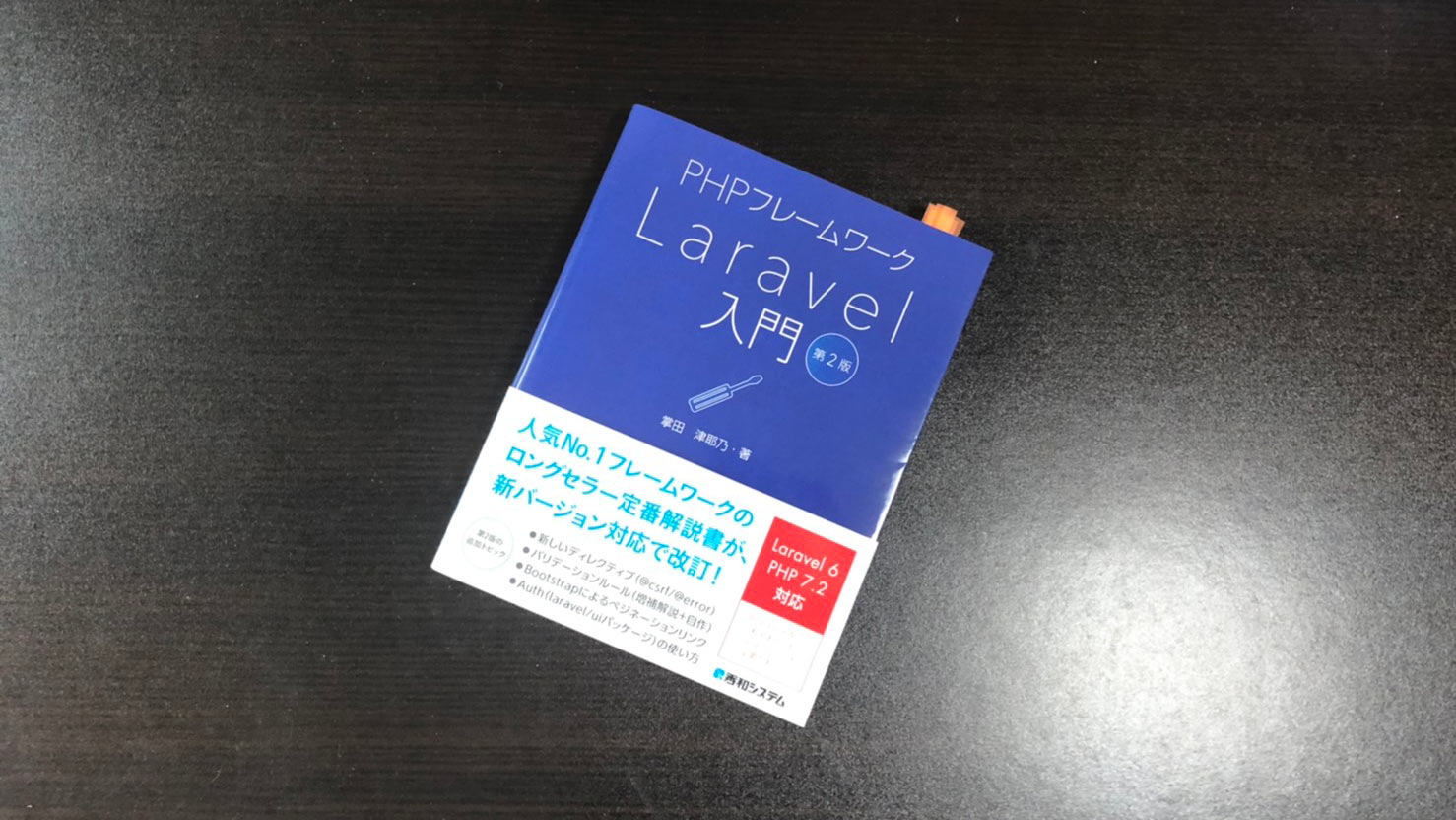